Azure App Services are a very easy and economical way to quickly deploy your ASP.NET Core apps to the cloud. You can get started for free if you just want to try out something you’re developing (without uptime considerations) and entry-level plans are pretty affordable:
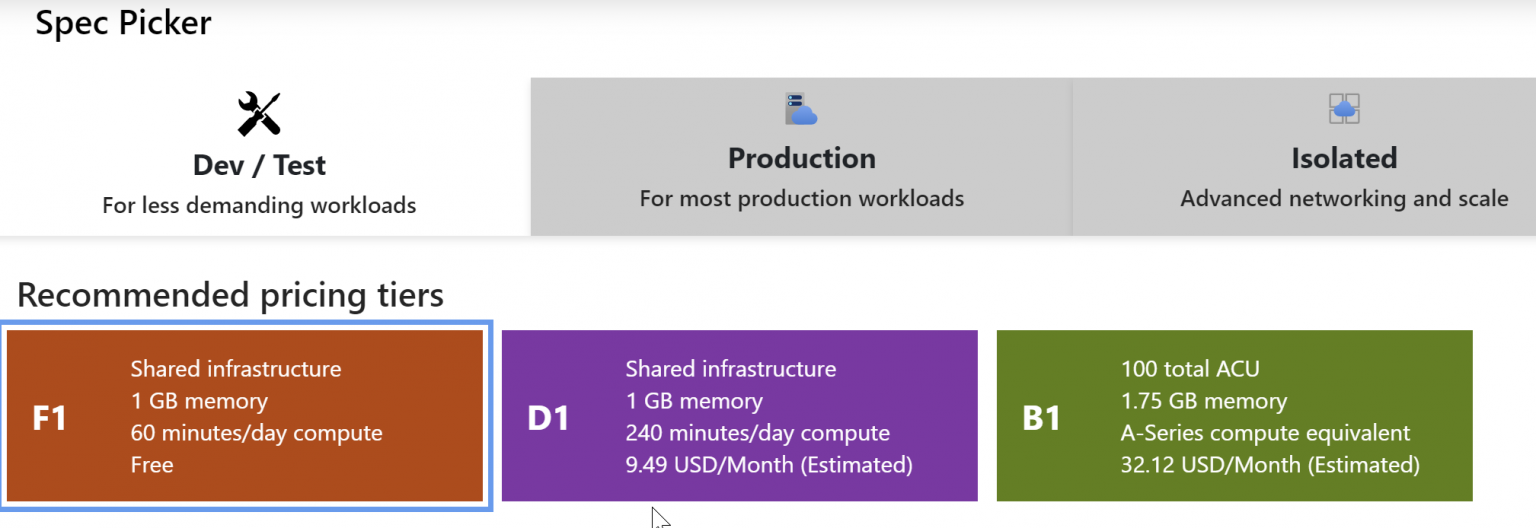
Ok, so now you’re publishing to an Azure App Service. But something’s not working quite right. How do you troubleshoot it? There are a variety of options. You can remote debug, but not at the lower-end shared hosting tiers shown above.
You can send an email, SMS, or other message for example. Another similar approach might be to use Application Insights to record when certain things occur.
I recommend that you configure logging, which is built-in with ASP.NET Core as a starter.
Configuring Logging to work with Azure App Service
The package you need is Microsoft.Extensions.Logging.AzureAppServices. Add it to your web project.
Now open your Program.cs and configure the logger as follows, adding any other loggers you might want beyond Console and AzureWebAppDiagnostics.
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder
.UseStartup<Startup>()
.ConfigureLogging(logging =>
{
logging.ClearProviders();
logging.AddConsole();
logging.AddAzureWebAppDiagnostics();
});
});
View Live Logs in Azure App Service
Once you have this configured, deploy your app to Azure App Service and you should be able to configure the log viewer to work. First, scroll down and click on ‘App Service logs’ and you should see something like this:
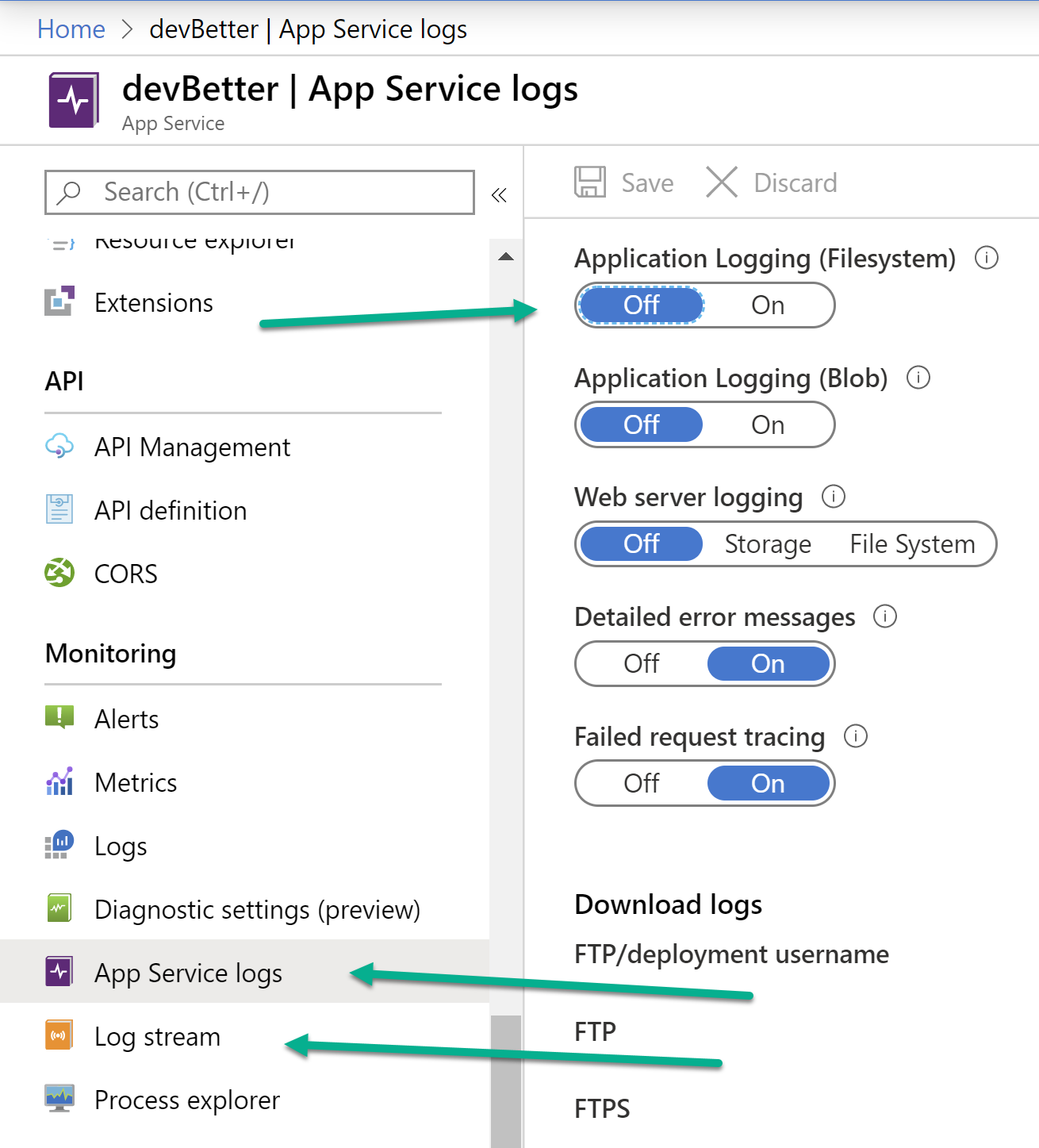
Configure Logging in Azure App Service
Next, on the right, toggle Application Logging (Filesystem) to enable it. This setting lasts for 12 hours and then automatically resets, to avoid filling your app’s hard drive. If you want more persistent logging, configure a Blob storage account to hold the logs.
When you enable logs, you’ll be able to set how verbose of messages you want to capture. If this is your first test, I recommend using Information so you’ll see all traffic to your site. Later, you might want to only look at Warnings or Errors. Save your selection.
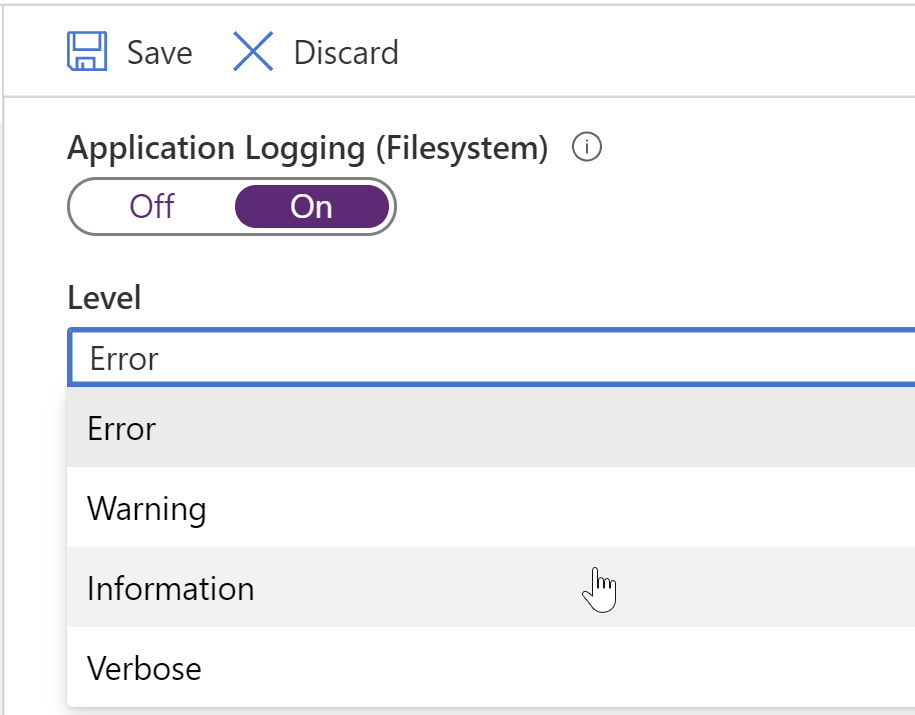
Choose Log Level.
Now click on Log stream on the left to attach to your application’s logs. Navigate to your site and you should see log messages corresponding to the requests you’re making to the application.
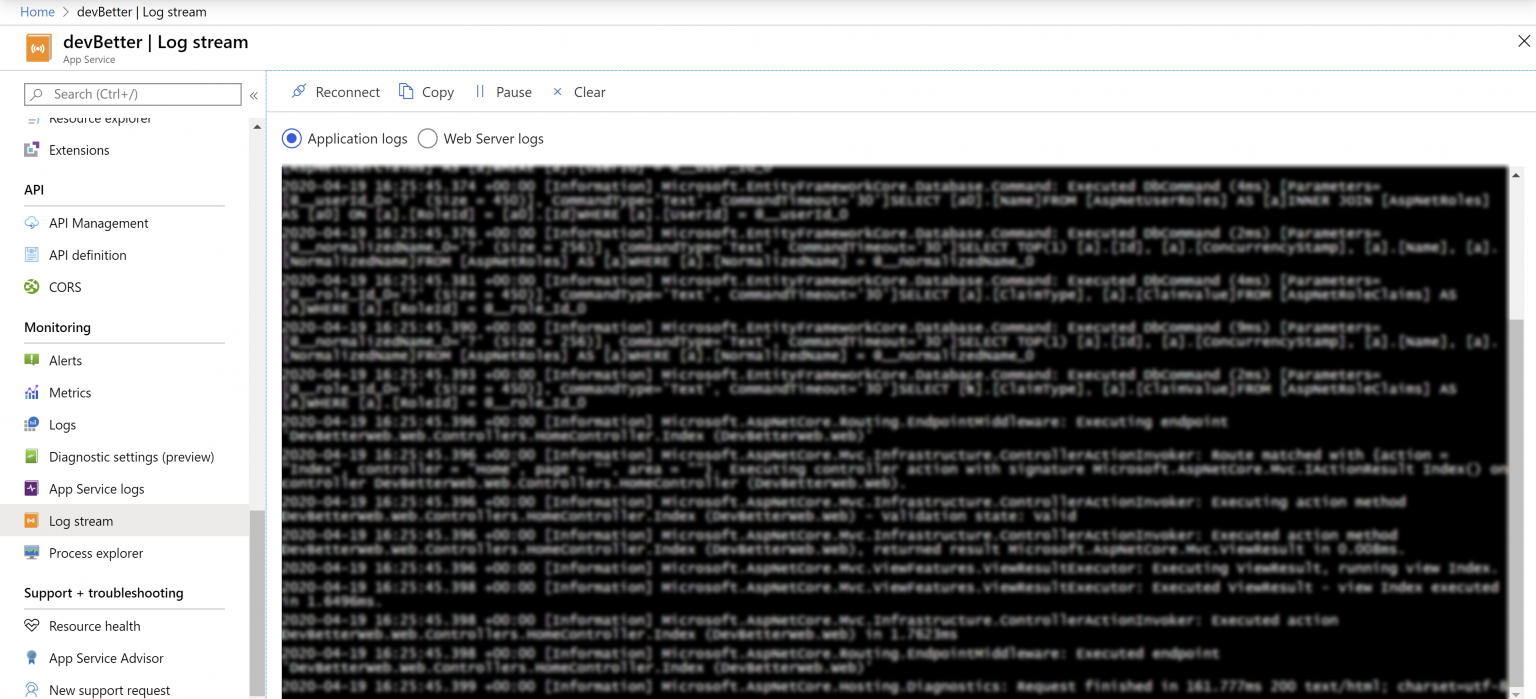
View your Azure App Service live log stream.